В этой статье посмотрим как можно отправлять электронные письма при помощи Python. Есть и более простые способы это сделать, но мне больше подошел именно следующий вариант.
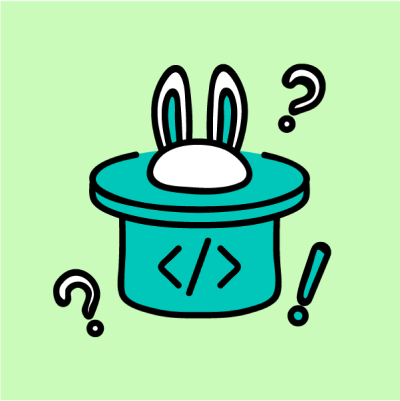
Отправка электронных писем с помощью Python
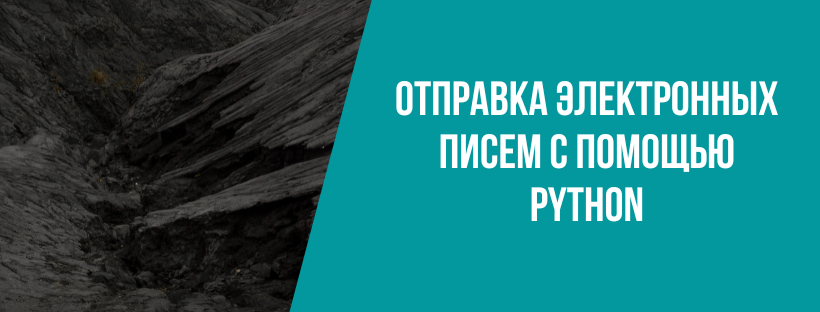
Итак, вот как это выглядит: у вас есть имена и адреса электронной почты некоторой группы контактов. И вы хотите отправить каждому из них письмо, добавив в начале сообщения «Уважаемый [имя]».
Для простоты вы можете хранить всю контактную информацию просто в файле, а не в базе данных. Также вы можете сохранить в файл шаблон сообщения, которое вы хотите отправить.
Модуль smtplib
в Python – это практически все, что вам понадобиться для отправки простых электронных писем без заполнения темы письма или какой-либо еще дополнительной информации. Но, конечно, для настоящих писем вам необходимо заполнить строку темы письма и другую информацию, и, возможно, даже прикрепить изображения или какие-то другие вложения.
Вот тут и приходит на помощь пакет email
в Python. Имейте в виду, что нельзя отправить сообщение через электронную почту, используя только этот пакет. Вам необходимо совместить email
и smtplib
.
Обязательно ознакомьтесь с подробной официальной документацией для каждого из этих пакетов.
Вот четыре основных шага для отправки электронных писем с помощью Python:
- Настройте SMTP-сервер и войдите в свою учетную запись.
- Создайте объект сообщения
MIMEMultipart
и загрузите его с соответствующими заголовками для полейFrom
(От),To
(Кому) иSubject
(Тема). - Добавьте тело сообщения.
- Отправьте сообщение с помощью объекта SMTP-сервера.
А теперь давайте рассмотрим весь процесс.
Допустим, что у вас есть файл контактов mycontacts.txt
, который выглядит вот так:
user@computer ~ $ cat mycontacts.txt john johndoe@example.com katie katie2016@example.com
Каждая строка соответствует одному контакту. В каждой строке пишется имя, а за ним следует адрес электронной почты. У меня все сохранено в нижнем регистре. Я оставлю преобразования любых полей или некоторых начальных букв в верхний регистр логике программирования, если это будет необходимо. В Python это все довольно просто.
Далее у нас есть файл с шаблоном сообщения message.txt
.
user@computer ~ $ cat message.txt Dear ${PERSON_NAME}, This is a test message. Have a great weekend! Yours Truly
Обратили внимание на ${PERSON_NAME}
? Это шаблонная строка в Python. Шаблонные строки можно легко заменить другими строками; в данном примере ${PERSON_NAME}
будет заменено настоящим именем человека (вы скоро это увидите).
А теперь давайте перейдем к коду Python. Для начала нам необходимо прочитать контакты из файла mycontacts.txt
. Мы, кстати, можем обобщить это в отдельную функцию.
# Function to read the contacts from a given contact file and return a # list of names and email addresses def get_contacts(filename): names = [] emails = [] with open(filename, mode='r', encoding='utf-8') as contacts_file: for a_contact in contacts_file: names.append(a_contact.split()[0]) emails.append(a_contact.split()[1]) return names, emails
Функция get_contacts()
в качестве аргумента принимает имя файла. Она откроет этот файл, прочитает каждую строку (то есть каждый контакт), разделит их на имя и адрес электронной почты, а затем добавит их в два отдельных списка. И, наконец, в качестве результата работы функции возвращаются эти два списка.
Также нам нужна функция для того, чтобы прочитать шаблон сообщения (такой как message.txt
) и вернуть объект типа Template
, созданный из его содержимого.
from string import Template def read_template(filename): with open(filename, 'r', encoding='utf-8') as template_file: template_file_content = template_file.read() return Template(template_file_content)
Как и предыдущая функция, в качестве аргумента она принимает имя файла.
Для того, чтобы отправить письмо, вам необходимо использовать протокол SMTP (Simple Mail Transfer Protocol – протокол простого обмена электронной почтой). Как уже упоминалось ранее, у Python есть необходимые для этого библиотеки.
# import the smtplib module. It should be included in Python by default import smtplib # set up the SMTP server s = smtplib.SMTP(host='your_host_address_here', port=your_port_here) s.starttls() s.login(MY_ADDRESS, PASSWORD)
В приведенном выше фрагменте кода вы импортируете smtplib
, а затем создаете экземпляр SMTP, который формирует SMTP-соединение. В качестве параметра он принимает адрес хоста и номер порта, оба эти параметра полностью зависят от настроек SMTP вашего поставщика услуг электронной почты. Например, в случае Outlook строка под номером 4 будет выглядеть вот так:
s = smtplib.SMTP(host='smtp-mail.outlook.com', port=587)
Для того, чтобы все работало, вам необходимо использовать адрес хоста и номер порта конкретно вашего поставщика услуг электронной почты.
MY_ADDRESS
и PASSWORD
– это две переменные, которые содержат полный адрес электронной почты и пароль от учетной записи, которую вы собираетесь использовать.
А теперь самое время получить контактную информацию и шаблон сообщения, используя функции, которые мы определили ранее.
names, emails = get_contacts('mycontacts.txt') # read contacts message_template = read_template('message.txt')
А теперь давайте отправим отдельное письмо каждому из этих контактов.
# import necessary packages from email.mime.multipart import MIMEMultipart from email.mime.text import MIMEText # For each contact, send the email: for name, email in zip(names, emails): msg = MIMEMultipart() # create a message # add in the actual person name to the message template message = message_template.substitute(PERSON_NAME=name.title()) # setup the parameters of the message msg['From']=MY_ADDRESS msg['To']=email msg['Subject']="This is TEST" # add in the message body msg.attach(MIMEText(message, 'plain')) # send the message via the server set up earlier. s.send_message(msg) del msg
Для каждого name
(имя) и email
(адрес электронной почты) (из файла с контактами) вы создаете объект MIMEMultipart
, настраиваете заголовки типов содержимого From
(от), To
(кому), Subject
(тема) как словарь ключевых слов, а затем прикрепляете тело сообщения к объекту MIMEMultipart
в виде обычного текста. Возможно, вам захочется прочитать документацию, чтобы узнать больше о других типах MIME
, с которыми вы также можете поэкспериментировать.
Также обратите внимание, что в строке 10 выше я заменяю ${PERSON_NAME}
фактическим именем, которое было извлечено из файла с контактами с помощью механизма шаблонизации в Python.
В данном конкретном примере я каждый раз удаляю объект MIMEMultipart
и создаю его заново при каждой итерации цикла.
Как только вы проделаете все это, то сможете отправить сообщение, используя простую удобную функцию send_message()
объекта SMTP, который вы создали ранее.
А вот и полный код:
import smtplib from string import Template from email.mime.multipart import MIMEMultipart from email.mime.text import MIMEText MY_ADDRESS = 'my_address@example.comm' PASSWORD = 'mypassword' def get_contacts(filename): """ Return two lists names, emails containing names and email addresses read from a file specified by filename. """ names = [] emails = [] with open(filename, mode='r', encoding='utf-8') as contacts_file: for a_contact in contacts_file: names.append(a_contact.split()[0]) emails.append(a_contact.split()[1]) return names, emails def read_template(filename): """ Returns a Template object comprising the contents of the file specified by filename. """ with open(filename, 'r', encoding='utf-8') as template_file: template_file_content = template_file.read() return Template(template_file_content) def main(): names, emails = get_contacts('mycontacts.txt') # read contacts message_template = read_template('message.txt') # set up the SMTP server s = smtplib.SMTP(host='your_host_address_here', port=your_port_here) s.starttls() s.login(MY_ADDRESS, PASSWORD) # For each contact, send the email: for name, email in zip(names, emails): msg = MIMEMultipart() # create a message # add in the actual person name to the message template message = message_template.substitute(PERSON_NAME=name.title()) # Prints out the message body for our sake print(message) # setup the parameters of the message msg['From']=MY_ADDRESS msg['To']=email msg['Subject']="This is TEST" # add in the message body msg.attach(MIMEText(message, 'plain')) # send the message via the server set up earlier. s.send_message(msg) del msg # Terminate the SMTP session and close the connection s.quit() if __name__ == '__main__': main()